WordPress Functions Cheat Sheet: The Quick & Handy Guide for Developers
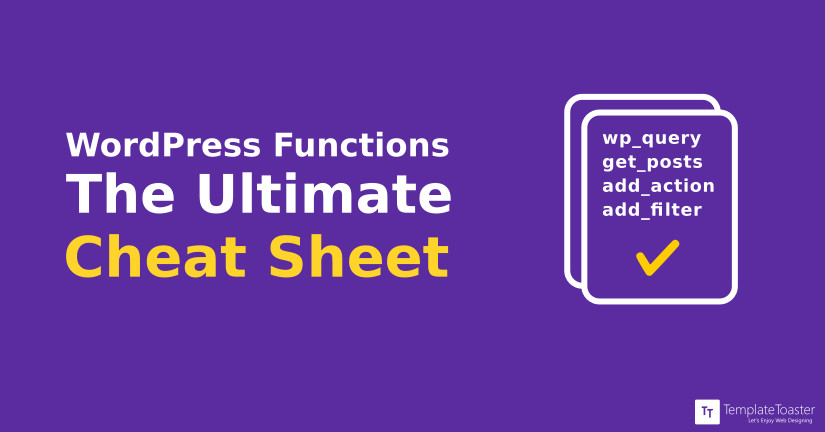
Looking for a quick and easy-to-use guide to commonly used WordPress functions? Use this handy cheat sheet to improve your skill and efficiency in WordPress plugin and theme development.
Most WordPress themes and plugins look for ways to create lists of posts based on multiple conditions and criteria. You often need to determine what you need on a page and pull posts accordingly. You may also need to use other information from the requests if you wish to create optimized pages. The WordPress database stores all data for posts, pages, comments, categories, tags, custom fields, users, site URLs etc. If we need more information on these then we need a way to extract this from the database. Writing direct SQL queries is not easy and not recommended. In this cheat sheet, we primarily look at the WordPress functions that are an easy and reliable way to work with the database.
WordPress Functions, Classes and More
WordPress functions provide the capability to create, modify WordPress themes and add more features to plugins. The number of WordPress functions is numerous and complicated. This makes it almost impossible to remember even the most basic functions. As such you might have become used to referring to the WordPress Codex Reference all the time.
You might have also wished that there was a quick and simple way around the wide array of functions and their capabilities. With this cheat sheet, we provide you with a listing of the most commonly used WordPress functions. The cheat sheet will guide you through the complexities of the WordPress functions and make coding simpler for you. We also show the usage of these functions with example PHP code.
1. WordPress WP_Query Class
The WP_Query is the class that describes a WordPress request. The class object provides details of a query request made by a post or page to a WordPress blog. It helps in determining the type of query like dated archive, feed, search etc. and accordingly fetch the posts.
You can use it to define a query for a page to filter the posts on a page. The WP_Query not only pulls specific posts for a page but also provides additional information about them. It is a safe, simple and modular approach for sending complex queries to the database.
Let us start with understanding how to create WP_Query objects. We will describe the commonly used WordPress functions to construct and use your own WP_Query objects.
WordPress new WP_Query function
You can create a WP_Query class object by calling the new WP_Query function. When creating the object, you can specify a list of arguments for it. These arguments define the WP_Query object and determine what posts will be there on the list in the object that you create.
The WP_Query() argument list is long and you can find them listed on its codex page.
Example to create a WP_Query object for all posts with tag “xyz” and the category “latest”:
//specify the argument list $args = array( ‘tag’ => ‘xyz’, ‘category => ‘latest’); //construct the query object $query = new WP_Query($args);
So here we see that we specify the arguments as an array stored in the variable $args. We then create a new WP_Query object with it and store the created object in the variable $query. This variable $query referencing can be done in the later code to retrieve the information.
WordPress defines various parameters which are sent as arguments to this function. Let us look at some of these parameters and the methods to create WP_Query objects with varying argument list.
WP_Query by id
The WP_Query object helps in retrieving the post or page by id using the ‘p’ and ‘page_is’ parameters respectively.
Display post by ID:
Specify the post id as the value of the parameter ‘p’ in the WP_Query argument list as shown below.
$query = new WP_Query( array( 'p' => 7 ) );
Display posts by page ID:
Specify the page id as the value of the parameter ‘page_id’ in the WP_Query argument list as shown below.
$query = new WP_Query( array( 'page_id' => 7 ) );
Sorting posts with WP_Query orderby
One of the commonly used arguments provided to the WP_Query() function is the ‘orderby’ parameter. You can use the order by argument to sort posts by parameters like title, author etc. One or more options are available for using in the argument. Also, here you need is the ‘order’ parameter which can be ascending or descending. If there is just a single option, it passes as the ‘orderby’ argument with ‘order’ value as ascending or descending. Multiple options can pass as an array of order parameters and order values. The default ordering parameter is the date (post_date) with the order as descending.
The example of a single orderby argument:
//order posts in descending order of title $args = array( 'orderby' => 'title', 'order' => 'DESC', ); $query = new WP_Query( $args );
The example of multiple orderby arguments with the same order:
//Display pages ordered by 'title' and 'menu_order’. $args = array( 'orderby' => 'title menu_order', 'order' => 'ASC', ); $query = new WP_Query( $args );
Note that the title is the higher priority and comes before the menu order in the order by list.
- The example of multiple orderby arguments with the different order:
$args = array( 'orderby' => array( 'title' => 'DESC', 'menu_order' => 'ASC' ) ); $query = new WP_Query( $args );
WP_Query Search Parameter
Use the search parameter to retrieve posts based on a keyword search. The parameter key is “s” and its value is the keyword to search.
Example to display posts that match the search term “search_item”:
$query = new WP_Query( array( 's' => ‘search_item’) );
The query object creates for different posts that match the keyword ‘search_item’ in search title and content.
WP_Query posts_per_page Parameter
The WordPress posts_per_page parameter for WP_Query specifies the number of posts to show per page. If you wish to show all the posts then use the value -1 for this parameter.
Example to Display 3 Posts per page
$query = new WP_Query( array( 'posts_per_page' => 3 ) );
Example to Display All Posts on a page
$query = new WP_Query( array( 'posts_per_page' => -1 ) );
There are other options available for this parameter which I am going to describe in detail in the codex reference.
How to use WP_Query?
The WP_Query class object creates when a page is loads in WordPress. Depending on the request URL, the object is able to access through the posts which depend on criteria like the first ten posts, post with the specific title, posts in a category etc.
There are two main scenarios when you can use the WP_Query object:
1. Determine the type of request: Use the WP_Query object to determine the request type for the current WordPress request. This information is present in the object properties named as $is_* properties and you shall retrieve it using these properties. This approach is generally done by using the plugin developers who want to add code to handle the types of requests.
A point to note here is that the $is_* properties are not directly in the code. Also, you can use this through Conditional Tags. The Conditional Tags helps in changing the content that shows up or displays. You can also define what content displays on a particular page depending on the conditions the page matches.
Examples of conditional tags:
WordPress is_home()
The is_home() function determines if the query is for the blog homepage. Use the is_home() conditional tag to display something on the home page of the blog.
The WordPress is_home() function specifies if the request is for the homepage of the blog. This function returns True if the blog view is on the homepage, otherwise false. Internally WordPress uses the get_option() function to retrieve the value of certain option values and check whether the current request is for the home page.
WordPress is_page()
The is_page() function determines if the query is for a page. This function has many variations to choose from, you may look at the reference for more details.
2. Go over multiple requests in sequence: The WP_Query object provides numerous functions for common tasks that are sequentially run for multiple posts. Within The Loop, you can process each post to be displayed on the current page, and format it based on specific criteria. You can add HTML or PHP code in the Loop that you want to process on each post. The two main WordPress functions for use in the Loop are the have_posts() and the_post() functions.
2. WordPress have_posts function
This have_posts() function is used to check if the current WordPress query has any results. If there are any results to loop over, then the function returns TRUE else it returns FALSE. You should normally use this function to check if your query returned the non-zero number of results.
$the_query->have_posts()
3. WordPress the_post function
Use the function the_post() to iterate the posts in the loop. This function uses an index to go over each post in the query results.
\$the_query->the_post()
These two functions are used in a while loop to sequentially carry out tasks for each post in the returned results.
<?php // Create the WP_Query object. $the_query = new WP_Query( $args ); //check to see if there are any results for the query if ($the_query ->have_posts() ){ while ($the_query ->have_posts() ) { $the_query ->the_post(); // Add code to display content for each post } }else{ // No posts found. } ?>
We have learned the basics of WP_Query and how to construct and use the query objects. Let us now move to somewhat advanced WP_Query functions.
4. tax_query WordPress function
The tax_query is used to perform queries based on taxonomies. A “taxonomy” is a grouping mechanism for posts, links or custom post types. When a taxonomy is created, it creates a special tax_query variable using the WP_Query class.
The tax_query takes an array of tax query arguments arrays. You can query multiple taxonomies by using the relation parameter to describe the Boolean relationship between the taxonomy arrays.
Let us look at a simple example to display posts tagged with the ‘Image’ term, under ‘content-type’ custom taxonomy:
$args = array( 'post_type' => 'post', 'tax_query' => array( array( 'taxonomy' => 'content-type', 'field' => 'slug', 'terms' => 'Image', ), ), ); $query = new WP_Query( $args );
If you want to explore more about how to use complex tax_queries then you can refer to the WP_Query reference.
5. WordPress the_excerpt
The WordPress the_excerpt() function displays the excerpt of the current post. It applies several filters to the excerpt including auto-p formatting.
This function doesn’t accept any parameters and trims the content to 55 words, and all HTML tags are stripped before returning the text.
Example usage for the_excerpt()
<?php the_excerpt(); ?>
6. WordPress the_title
The WordPress the_title() function displays or returns the title of the current post. Use this function from within The Loop and use get_the_title to get the title of a post outside of The Loop.
This function accepts as parameters the text to place before or after the title and whether the title should be displayed or returned for use in PHP.
Example usage for using the_title() to retrieve the post title in a “Heading 3” format.
<?php the_title( '<h3>', '</h3>', FALSE ); ?>
7. WordPress get_pages
The WordPress get_pages() function returns an array of pages that are in the blog. The function can also retrieve other post types using the ‘post_type’ parameter.
Example usage for get_pages()
<?php $args = array( 'sort_order' => 'asc', 'sort_column' => 'post_title', ); $pages = get_pages($args); ?>
8. WordPress the_category
The WordPress function the_category () displays a link to the category or categories a post belongs to. This function is also used from within The Loop.
The function takes as parameters the post id for which to display categories. It also takes in the separator to use for the categories and whether to show single or multiple links to child categories.
Example usage for the_category()for categories separated by a comma.
<?php the_category( ', ' ); ?>
9. WordPress add_filter
The WordPress add_filter function helps to hook a function or method to a specific filter action. The WP_Query Class supports around 16 WordPress filters. These are filters run by the WP_Query object when building and executing a query that retrieves posts. You can use the add_filter function to add a WP_Query filter for specific query actions.
add_filter( 'post_limits', 'my_post_limits_function' );
Here the ‘my_post_limits_function’ function is user-defined and you can add your code to modify the behaviour of the LIMIT clause of the query. An example would be to add a limit to the number of results returned in the WP_QUERY object depending upon if it is a search query on a search page.
There are many more filters available for use with WP_Query which you can use to modify various clauses of the query that returns the posts array. A few of these are mentioned below:
- posts_distinct
- posts_groupby
- posts_join
- post_limits
- posts_orderby
- posts_where
10. WordPress add_action
The WordPress add_action function helps to hook a function on to a specific action. Actions are the hooks that are launch at specific points during execution, or when specific events occur.
The WP_Query object also provides support for add_action(). With the ‘the_post’ action hook you can modify the post object immediately after it is queried.
//define action_hook function my_the_post_action( $post_object ) { // modify post object here } //register action_hook add_action( 'the_post', 'my_the_post_action' );
The pre_get_posts hook is called after the query object is created, but before the actual query is run. So, you can use it to change WordPress queries before they are executed.
You can refer to the codex reference for details on more actions supported by WP_Query objects.
11. WordPress get_posts function
The WordPress get_posts() function takes an ID of a post and returns the post data from the database. This function returns an array of WP_Post objects where each object represents an individual post which can be a post, a page or a custom post type.
The advantage of using get_post() over WP_Query() is that the main loop does not get altered which is not the case with using WP_Query(). Internally the get_posts() function also uses the WP_Query() object to retrieve posts based on the passed arguments. However, it ensures to reset the main loop which you might forget to do if you use WP_Query directly.
Here is a small example of how to use the get_posts() function:
$args = array( “post_type” => “post” ); $posts_array = get_posts($args);
In this case, we are getting an array of posts from the call to get_posts(). If you look at the function reference on the codex, there are many more parameters like posts_per_page, paged, tax_query etc.
WordPress get_posts custom post type
You can also use the get_posts() function to retrieve posts of a custom post_type with a custom taxonomy name
$args = array( 'post_type' => 'post', 'tax_query' => array( array( 'taxonomy' => 'content-type', 'field' => 'slug', 'terms' => 'Image', ), ), ); $posts_array = get_posts($args);
get_posts wpml
The WordPress Multilingual plugin adds support for multiple languages in WordPress pages. It also provides functions that work with the WordPress WP_Query and WP_Post classes and provide a way to retrieve and manage posts from specific languages.
The get_posts() function will by default return all posts for every language. To return only posts for the current language, you can follow any of the two approaches.
Use ‘suppress_filters’:
Set the ‘suppress_filters’ parameter of the get_posts function to false. This will enable the get_posts() function to only return posts in the current language.
$args = array( // add other arguments here 'suppress_filters' => false ); $posts_array = get_posts( $args );
WordPress query_posts function:
The other preferred option is to return posts using the query_posts() function instead. . It takes as a parameter an array of WP_Query arguments and returns a list of post objects. The query_posts()function alters the main query that WordPress uses to show posts. It replaces the main query with a new query. Therefore it requires as to call wp_reset_query() after query_posts() to restore the original main query//create arguments array with language set as en_us
$args = array( 'order' => 'ASC', 'lang' => 'en_us'); // use query_posts to retrieve posts in English. query_posts( $args );
It is important to note that using this function to replace the main query on a page can increase page loading times. This is not a recommended option and is also prone to confusion.
There are some other parameters that generally help with the query_posts() functions. The WordPress query_posts limit of posts to return that can be set with the ‘posts_per_page’ parameter. If you want to return posts from a specific page, then you can also use the query_posts ‘paged’ parameter. The WordPress query_posts offset parameter helps to specify the number of posts you want to skip before starting to list posts.
12. WP_Query post meta
The WP_Query post carries additional post information known as metadata. This meta-data can include bits of information such as Mood, Weather, Location etc.
The Meta-data stores as key/value pairs. The key is the meta-data element name and the value is the information. The value is shown in the meta-data list of any post the information is linked to.
There are few WordPress functions for WP_Query post meta to add, remove, retrieve meta information.
WordPress get_post_meta
The get_post_meta function retrieves the post meta field for a post.
get_post_meta($post_id, $key, $single);
As seen above, we pass as arguments the post id, the meta key and whether we want a single result as a string or an array of fields.
meta_value WordPress
The meta_value WordPress function allows you to get a post with a selected meta key and meta value.
As an example, we show how to filter posts with the meta_key set as ‘show_on_firstpage’ and meta_value as ’on’.
$rd_args = array( 'meta_key' => 'show_on_firstpage', 'meta_value' => 'on' ); $query = new WP_Query( $rd_args );
13. WordPress WP_Meta_Query Class
The WP_Meta_Query Class is the core class used to implement Meta queries for the Meta API. You can use this class object for generating SQL clauses to filter a primary query as per metadata keys and values. WordPress stores three kinds of metadata post meta, user meta, and comment meta. You can consider this class as a helper to primary query classes, such as WP_Query, used to filter their results by object metadata.
To construct an object of the WP_Meta_Query class you can pass several arguments as a key=>value paired array. The available keys that this function takes as arguments are:
- meta_key
- meta_value
- meta_type
- meta_compare
An example of simple usage of the constructor to create an object of the WP_Meta_Query class is given below.
$meta_query_args = array( array( 'key' => 'custom_key', 'value' => 'custom_value' )); $meta_query = new WP_Meta_Query( $meta_query_args );
WordPress meta_query compare
At times, you may want to compare the values corresponding to metadata keys. The default mechanism for comparing meta_values is the ‘=’ operator that checks for equality. If you want to choose from other options like ‘! =’, ‘>’, ‘<’ etc. then you may use meta_query compare argument. You should provide it as the compare argument in the arguments array.
The possible values are ‘=’, ‘!=’, ‘>’, ‘>=’, ‘<‘, ‘<=’, ‘LIKE’, ‘NOT LIKE’, ‘IN’, ‘NOT IN’, ‘BETWEEN’, ‘NOT BETWEEN’, ‘EXISTS’ (only in WP >= 3.5), and ‘NOT EXISTS’ (also only in WP >= 3.5). Values ‘REGEXP’, ‘NOT REGEXP’ and ‘RLIKE’ were added in WordPress 3.7
$meta_query_args = array( array( 'key' => 'custom_key', 'value' => 'custom_value' 'compare' => 'EXISTS', )); $meta_query = new WP_Meta_Query( $meta_query_args );
Final Thoughts
The WordPress developers have access to an extensive list of WordPress functions to customize their themes. We have put together a list of the most commonly used WordPress Query functions. However, we all know that learning to use these functions may prove to be time-consuming in the beginning. Developers tend to look for options that are easy to use and give them similar functionality and flexibility.
WordPress is a powerful CMS platform with an amazing templating engine that the developers can use to create beautiful designs for the platform. However, many times you may want to customize and change the theme which would need coding. For this, you need to do some basic PHP, HTML, and CSS.
However, before that, it is always better to know and understand how WordPress works on the backend and then start with the themes and templates.
TemplateToaster, a WordPress theme builder and WordPress website Builder is one such tool with advanced features and functionality for creating WordPress themes smoothing the development work considerably. The tool is a comprehensive web design software that provides support for all WordPress plugins and customized themes. With this tool, you will not need to delve into the details of WordPress functions and can directly use themes from the vast collection that TemplateToaster offers.
[call_to_action color=”gray” button_icon=”download” button_icon_position=”left” button_text=”Download Now” button_url=”https://templatetoaster.com/download” button_color=”violet”]
Best Drag and Drop interface to Design stunning WordPress Themes
[/call_to_action]
Build a Stunning Website in Minutes with TemplateToaster Website Builder
Create Your Own Website Now
Nice tricks…. Greetings from sntechno.com
Once the developer done their work, have a look at these, this may help in checking the mistakes or bugs.