How to Create WordPress Theme from Scratch: Step by Step Guide
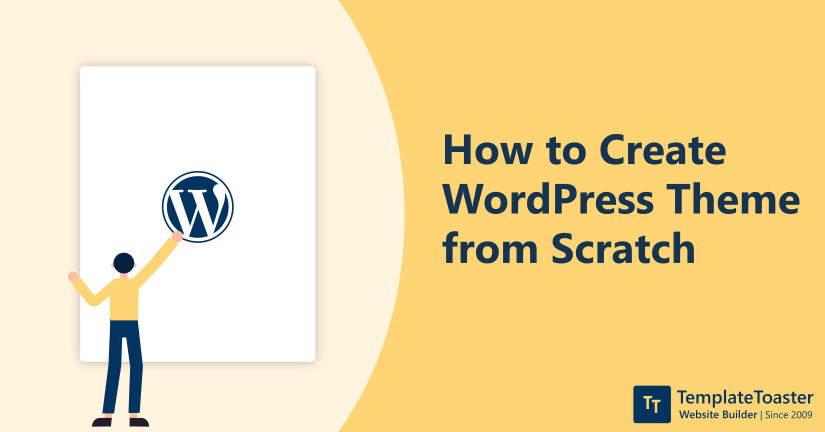
Creating WordPress theme from scratch can be really simple if you know basic HTML, CSS, and JavaScrip. However, if you are new to WordPress, all you have to do is to follow the steps mentioned in this tutorial for beginners. However, if you are new to WordPress or a beginner, do not worry, you can even build a theme for WordPress now. Here I have listed simple steps that will help you create your own WordPress theme from scratch in a breeze. So, let’s read this tutorial guide and learn how to create WordPress theme from scratch.
WordPress is a widely used open-source content management system (CMS) around the globe. You can also choose this platform to create a strong online presence and establish your business online effortlessly with the help of powerful WordPress software. WordPress is an obvious choice and the simplest one to begin with. And that is the main reason why every developer suggests choosing it as the base of your site. However, if you are still not sure about what is WordPress then reading this guide will definitely shed some light to make things clear for you.
So, without much ado, let’s begin with developing WordPress theme step-by-step process
How to Create a WordPress Theme? Tutorial for beginners
Designers and developers have been unconsciously partial towards WordPress based themes for not just one but several reasons. At times, some client specifically asks for a WordPress site. And the main reason behind the huge popularity of WordPress lies in its simplicity. In fact, it is a highly flexible and powerful CMS. Hence, those who are working with any other CMS(s) like Joomla, Drupal, etc. may sometimes wish to migrate i.e. from Joomla to WordPress or so on. So, let’s now understand why people love this platform so much.
What are the Requirements to Create WordPress Theme?
1. Installing WordPress Locally
First thing first, you need to install WordPress. Don’t worry it’s not rocket science to learn how to install WordPress on Localhost. Now open XAMPP and start Apache and MySQL. You can easily do that yourself.
2. Procedure to create a WordPress Theme From Scratch.
- Manual – creating WordPress theme via coding
- Automated – creating WordPress theme using a WordPress Theme Builder
For WordPress theme, everything will be done in the wp_content directory only. Just make a new theme subfolder in the wp_content → Themes folder. Let’s assume you name it “mytheme”.
The second thing is to decide the layout of the theme. Here, the tutorial is showing the basic layout consisting of Header, Main Area, Footer, Sidebar.
Basically, WordPress needs only 2 files i.e. style.css and index.php. But for this layout, you need 5 files, as follows;
- header.php – contains the code for the header section of the theme.
- index.php – contains the code for the Main Area and will specify where the other files will be included. This is the main file of the theme.
- sidebar.php – contains the information about the sidebar.
- footer.php – handles the footer section.
- style.css – responsible for the styling of your theme.
- bootstrap.css – no separate CSS code is required; highly responsive.
- bootstrap.js – provides its own js for the navigation bar, or tabs, etc.
You need to download the Bootstrap 5 package. Bootstrap.js & Bootstrap.cs file needs to be copied to the theme folder.
How to Create Custom WordPress Theme step by step?
Create WordPress Theme from Scratch by Coding (Manually)
Create WordPress Theme with TemplateToaster (Automated)
You can create these files locally with a text editor like Notepad. Below are the files, you need to create to get started.
Steps for Creating WordPress Theme from Scratch by Coding
Step 1: header.php File
You need to put this code in the header.php file.
<html> <head> <title>Tutorial theme</title> <script type="text/javascript" src="<?php echo get_stylesheet_directory_uri().'/js/jquery.js'; ?>"> </script> <script type="text/javascript" src="<?php echo get_stylesheet_directory_uri().'/js/jquery-ui.min.js'; ?>"> </script> <script type="text/javascript" src="<?php echo get_stylesheet_directory_uri().'/js/bootstrap.js'; ?>"> </script> <link rel="stylesheet" href="<?php echo get_stylesheet_directory_uri().'/css/bootstrap.css'; ?>"> <link rel="stylesheet" href="<?php bloginfo('stylesheet_url'); ?>"> </head> <body> <div id="ttr_header" class="jumbotron"> <h1>HEADER</h1> </div> <div class="container">
This file Header.php contains the code for the header part in which the js and style file is linked. It displays the header of the page.
<link rel="stylesheet" href="<?php bloginfo('stylesheet_url'); ?>">
This line added after the title tells WordPress to load a style.css file that will handle the styling of the theme.
Here,
<?php bloginfo('stylesheet_url'); ?>
is a WordPress function that actually loads the stylesheet.
<link rel="stylesheet" href="<?php echo get_stylesheet_directory_uri().'/css/bootstrap.css'; ?>">
Here, we use Bootstrap. It is one of the well-known responsive web design frameworks. It provides inbuilt CSS files to style your site. You can keep the bootstrap.css file in your theme/css folder.
Next, a “div” with class ttr_header is added and this will be the main container of the site. Now, set a class for it so that you can modify it via the style.css file.
After that, add a simple label HEADER in a “div id” with class “ttr_header” which will be later specified in the class “jumbotron”.
Step 2: index.php File
The main file index.php will contain the following code;
<?php get_header(); ?> <div id="ttr_main" class="row"> <div id="ttr_content" class="col-lg-8 col-sm-8 col-md-8 col-xs-12"> <div class="row"> <?php if (have_posts()) : while (have_posts()) : the_post(); ?> <div class="col-lg-6 col-sm-6 col-md-6 col-xs-12"> <h1><?php the_title(); ?></h1> <h4>Posted on <?php the_time('F jS, Y') ?></h4> <p><?php the_content(__('(more...)')); ?></p> </div> <?php endwhile; else: ?> <p><?php _e('Sorry, no posts matched your criteria.'); ?></p> <?php endif; ?> </div> </div> <?php get_sidebar(); ?> </div> <?php get_footer(); ?>
The very first line of code in this file
<?php get_header(); ?>
will include the header.php file and the code in it are on the main page.
<?php if (have_posts()) : while (have_posts()) : the_post(); ?> <div class="col-lg-6 col-sm-6 col-md-6 col-xs-12"> <h1><?php the_title(); ?></h1> <h4>Posted on <?php the_time('F jS, Y') ?></h4> <p><?php the_content(__('(more...)')); ?></p> </div> <?php endwhile; else: ?>
The above code displays the main content of the post that you have created through the WordPress administrative area.
Next, add the sidebar.php file like shown below
<?php get_sidebar(); ?>
In this file, you can display your recent posts, archives, contact info, etc.
After this line, an empty “div” inserted that will separate the Main Area and the Sidebar from the footer.
Finally, added one last line
<?php get_footer(); ?>
which will include the footer.php file.
Step 3: Sidebar.php File
In the sidebar.php, add the following code
<div id="ttr_sidebar" class="col-lg-4 col-md-4 col-sm-4 col-xs-12"> <h2 ><?php _e('Categories'); ?></h2> <ul > <?php wp_list_cats('sort_column=namonthly'); ?> </ul> <h2 ><?php _e('Archives'); ?></h2> <ul > <?php wp_get_archives(); ?> </ul> </div>
In this file, internal WordPress functions are called to display the different Categories, Archives of posts. The WordPress function returns them as list items, therefore, you have to wrap the actual functions in unsorted lists (the <ul> tags).
Step 4: footer.php File
Add the below code lines to the footer.php file:
<div id= "ttr_footer"> <h1>FOOTER</h1> </div> </div> </body> </html>
This will add a simple FOOTER label. However, you can also add links, additional text, the copyright information for your theme in place of plain Footer text.
Step 5: style.css File
Add the following lines to the style.css file
body { text-align: left; } #ttr_sidebar { border-left: 1px solid black; } #ttr_footer { width: 100%; border-top: 1px #a2a2a2 solid; text-align: center; } .title { font-size: 11pt; font-family: verdana; font-weight: bold; }
This CSS file sets the basic appearance of your theme. This will set the background of the page and add the borders as per your needs.
Your page shall look somewhat like this:
Now, you can further modify the CSS file by adding images, animations and other content to your theme and make it look as beautiful as you want.
But, this will require the HTML, PHP and WordPress functions knowledge. Thus, a better alternative is to use a strong WordPress Theme Builder that doesn’t include even a single code line. Yes! Without coding! A software that lets you create your own WordPress theme from scratch without any coding knowledge.
Eager to know about that solution, well, I am talking about your very own theme builder software TemplateToaster. It provides an array of fantastic features with an easy to use drag & drop interface. So, without wasting a minute, let’s begin developing WordPress theme from scratch with TemplateToaster.
How to Create WordPress Theme with TemplateToaster?
TemplateToaster is pretty easy to install and it doesn’t involve any coding at all. Simply visit the software website and download the TemplateToaster installer. However, the trial version is free. Now, all you have to do is follow these easy steps to make your own WordPress theme. The first screen that will appear after installation looks like
Follow These Steps to Create WordPress Theme & Initiate your WordPress Theme Development
Step 1: Choose a Platform
The first screen that will appear after installation looks like the following. Here, you can make the CMS selection. Since we are creating a WordPress theme so the obvious choice here should be WordPress.
Now you can see a screen with two options i.e. Go with Sample Templates and Start from Scratch. You can easily find one suitable template from the plethora of free WordPress themes. Since we are involved in WordPress template development thus, I will choose “Start from Scratch”.
Now, you shall see the very first pop-up asking you to select Color and Typography for your WordPress theme from the given options. Click on the OK button to confirm your choice.
Step 2: Design a Header
Here you need to select the width and height of the header. You can keep the width to full width, equal to container width, and custom width can also be set.
Next, you need to select the Background Color for your header. You can set a color, gradient, or you can also browse an image either from the built-in image gallery or you can use your own custom image.
Now, you can add ‘Text Area’ to your header and add text of your choice.
Next, you can easily add Social Media Icons and make your header as interactive as you want.
The Header is ready now.
Step 3: Design Menu
Now, is the time to design the Menu. Go to the Menu tab and select the width and height you want to set for your Menu from the given options.
Similarly, you can set the background for your menu. You can select from the given options such as Color, Gradient, and Images.
Now, select the Logo for your Menu. Go to the logo tab and choose any logo if you find any suitable logo from the gallery. Otherwise, you can choose your own custom logo as well. Just go to the browse option and look for your logo and have it on your Menu. It’s important to choose the color, font, and shape for your logo according to your business goals and services.
In order to set the Menu Buttons, head to the ‘Menu Button Properties’ and then align the button like Alignment → Horizontal → Right to Page. This will align your menu buttons to the right of the page.
However, you can also set the typography for your menu. Simply by going to the Typography → Normal/Hover/Active. Whereas, there is an option to change text color as well.
Step 4: Create and Stylize a Slideshow
In order to add a slideshow, go to the Slideshow tab and check the slideshow option provided at the left extreme corner. You need to check the checkbox and it will add a slideshow to your WordPress theme.
There are options to set the position of the slideshow such as below, above, or middle. Likewise, you can set the height and width of the slideshow so that it should match with other elements available on your WordPress theme.
You can select what Background Color and Background Image you want for the slideshow. Click on the background option and you are free to select images either from the built-in gallery or you can choose your custom images too.
Further, you can easily stylize it by applying properties like the position of the slideshow, transition effects, width, height, border, etc., and make it as beautiful as you want. You can then add a text area to display the required information.
These and many other components top WordPress developers include in their theme to make them more engaging for users.
Step 5: Edit Your Content (Main Area)
Now comes the main area i.e. Content area. Simply by clicking on the text, you will be able to add the content to your site. However, you can use various options like you can set the typography, font color, text alignment, font size, video, images, contact form, tables to your columns, and much more. This way you can get your text ready. Because content plays a significant role. You can find and engage your target audience with content.
Step 6: Design/Customize the Footer
In order to design your Footer, go to the Footer tab and start picking up the options to customize it. You will get to see many options like setting the Background Image or color, Typography, Margin, Padding, Height, Width, etc. You can also have social icons in your footer, links, hyperlinks, and much more.
Step 7: Add More Pages
So, you have successfully designed the home page. Similarly, you can create other pages too. All you have to do is click on the ‘+’ icon on the left side and add as many pages as you want. When you will click on the + icon, a pop-up will appear where you have to enter your Name, Title, Slug, and then click on the Save button to confirm your action. This way you can add as many pages as you want.
However, if you want to add a child page for any particular page, you can do that as well. All you have to do is click on the ellipses (three dots) corresponding to the page name and then right-click being present on the desired page and choose the ‘Add Child Page’. Something like showing a virtual hierarchy.
Page Name → ⋮ → Add Child Page
Here, you will see other options such as Remove, Edit, and Clone. You can use options to take suitable actions.
Step 8: Export Your WordPress Theme
After you have successfully created your WordPress theme, now you need to export it. Go to the File tab and click on the ‘Export’ option there.
After you click on it, you shall see an ‘Export WordPress Theme’ pop-up. Here you need to add the file name and then browse the path where you wish to export your WordPress theme. Once you are done, hit the Export button.
Step 9: Log in to Your WordPress Admin Panel
Now, login to your WordPress dashboard and go to Appearance → Theme → Add New
There’s an option given ‘Upload Theme’. Click on this option to upload your theme to WordPress.
Browse your WordPress theme (it will be a zip file) and hit the Install Now button to confirm your choice.
You shall now see a screen with the message ‘Installing Theme from uploaded file: yourfilename.zip’. Here, you need to click on the Activate button and this will activate your installed theme.
However, you have created your WordPress theme with TemplateToaster, it provides a default Contact Form 7 integration. Therefore, it will display a message informing you about the theme you have created requires Contact Form 7 installed. So, click on the ‘Begin installing plugin’ option and let the plugin install for your WordPress.
Since TemplateToaster comes with the ease to add content to your theme. So, whatever content you have added to your WordPress theme while creating it can be directly exported here. All you have to do is go to
Appearance → Theme Option → Import Content Tab → Import Content Button
Now, there appears another pop-up the ‘Import Content’ pop-up. Here you can choose what content you wish to import. Pages, Menu, Footer Menu, all the options are given and you are free to choose which page or what content you wish to show. However, you can select all options and let all of your content be imported all at once.
After all the steps you are now ready with your WordPress theme. It will look something like the below screenshot.
Hurry! Your theme looks beautiful. I am sure all these steps are now clear to you and you are ready to create your own custom WordPress theme using TemplateToaster.
However, if you want to add a child page for any particular page, then you can do that as well. And all you have to do is click on the ellipses (three dots) corresponding to the page name t have to right-click being present on the desired page and choose the ‘Add Child Page’. Something like showing a virtual hierarchy.
Page Name → ⋮ → Add Child Page
Here, you can see other options also i.e. Remove, Edit, and Clone. You can use them to take suitable actions.
Hurray!! You have completed process of creating WordPress theme form scratch. And you can earn profit with WordPress theme development and keep your little secret weapon safe.
TemplateToaster, web design software offers many more advanced options like putting a Video background, slideshows, new menu styles, etc. You can learn more about how to create professional themes and templates, and how to use WordPress theme check plugin so on.
Which Method You Use for Creating WordPress Theme?
So, this brings us to the end of this comprehensive tutorial. I am sure it will help you build your own WordPress theme, also explain the primary aspects of creating WordPress theme from scratch. Creating WordPress theme is quite simple and the choice of CMS depends on your purpose. Like if you wish to create a child theme in WordPress or you may even want to know what WordPress theme is that, or you want to begin WordPress theme development using Bootstrap, etc. There are certain things to keep in mind before you choose WordPress theme because choosing the best WordPress theme is no child’s play. Check out best WordPress themes and free WordPress themes.
Conclusion of WordPress theme creation
Well, creating your own custom WordPress theme from scratch is that easy, especially if you are using WordPress theme builder. Whether you want to create a personal or business website you can create custom WordPress themes without any coding knowledge. In this article, I’ve tried my best to cover every detail on creating WordPress themes for beginners, without writing any single line of code. Hopefully, now the creation process is simpler for you and you can try out generating new WordPress themes from scratch. However, if you still have doubts or any questions in mind, do let me know in the comment section.
Build a Stunning Website in Minutes with TemplateToaster Website Builder
Create Your Own Website Now
I am glad you wrote this to give alternative ways. My one complain about Template Toaster has always been that I cannot submit themes created with it to the official WordPress repos. I really hope your team will resolve the issues preventing that.
This article obviously took significant time to put together. Very well written, very clear, very helpful. Nice work!
In the sidebar.php you have an unopen tag: me&optioncount=1&hierarchical=0′); ?>
What’s the rest of it?
Hi Jeff, we are sorry for that. It was a human error. We have updated the code. Thanks for pointing out.
Is it possible to add a menu? And if so, how?
Is it possible to add custom login plugin when creating WordPress theme from scratch in TemplateToaster?
And Thanks for sharing this informative post!
yes of course, you can add.
Plugins like Admin Custom Login plugin, Loginer, ThemeMyLogin works fine.
I just installed TemplateToaster and came across this article. Really helpful.
Created a WordPress theme from scratch successfully. Thanks !!
How can i have swiping images in the home page?
Good day! I could have sworn I’ve been to this
blog before but after reading through some of the post I realized it’s new to me.
Nonetheless, I’m definitely delighted I found it and I’ll be book-marking and checking back frequently!
Creating a WordPress is not a tough nut to crack but still you can get stuck anywhere and need some guidance. Very Impressive post. Thanks for sharing 🙂
Best post witnessed for WordPress theme from scratch!
Thanks TemplateToaster blog.
Very True! I have been looking for this kind of blog.
And Creating a WordPress theme with TemplateToaster is also very easy. Thanks TemplateToaster blog.
Indeed an impressive post!
All the information at one place. WordPress is becoming easier and easier day by day with these kind of blogs.
Do you know any WordPress custom login page plugin ?
Loginer is a good WordPress plugin to create login page
Thanks for replying, I am already using it, Good plugin
I was regularly getting some error in the script. Your blog came to me as rescue.
Thanks for sharing.
This post must have taken a great time and is totally appreciable.
Creating a theme from scratch is not easy , a single error can drive you crazy.
Thanks for sharing the post.
Very true, front end development is also a tough task since this makes most impact on the visitor of the website. This post is very helpful
Very impressive post 🙂
Please recommend me a WordPress plugin for email newsletter.
You could try MailChimp for email marketing and newsletter services.
This post is a Gem for WordPress lovers!
Looking for it from a long time.
WordPress theme from scratch is a good option but also modifying the pre-designed templates can also get your work done.
hi , which editor would you recommend to modify a Premium WordPress theme?
You guys always come up with great content. This article is really helpful and must have taken good amount of time to put it together.
How can i add a video in the home page of the website using TemplateToaster?
Best post ever witness on WordPress theme from scratch. Thanks for sharing.
Indeed a great post. WordPress theme from scratch is really tough but with time and practice finally got success.
Yes, clearly explained. Thanks for sharing.
How can i design a corner ad in the home page?
TemplateToaster is a great platform to create WordPress templates. Thanks for the article.
Does TemplateToaster’s free trial provide this feature of creating the theme from scratch?
No, Only its professional edition has this feature of creating Themes from scratch.
How can I add custom Images in TemplateToaster?
Creating from scratch is not a cake walk, thanks for sharing the post.
Great post! Thanks for the complete tutorial, very well explained
Professional web designers can use TemplateToaster to create WordPress themes from scratch doing all the customizations with tge WordPress theme builder .
Which WordPress version you are using in your example ?